Param
While working with Robocut Studio building custom LED controllers I had added a interactive parameter system to handle device configuration and operation. It was clear it should be its own library so I refactored it as a Arduino library for devices with wired or wifi network connections. It is particularly useful in a creative process where new parameters can be quickly added and manipulated in real-time.
Features:
- Various data type parameters:
- bang
- boolean
- integer
- float
- string
- enumeration
- color
- OSC style addresses
- compatibility with OSC
- no extra parsing code needed
- defines nested menu structure
- Websocket server + web interface
- webparam is a static webpage where you enter a Param device IP
- device pushes all of it's parameters back
- webparam builds a nested interface according to parameter addresses
- save and load as JSON
- uses ArduinoJSON
- save parameters to SDcard or flash
Find out more at https://gitlab.com/maxd/param
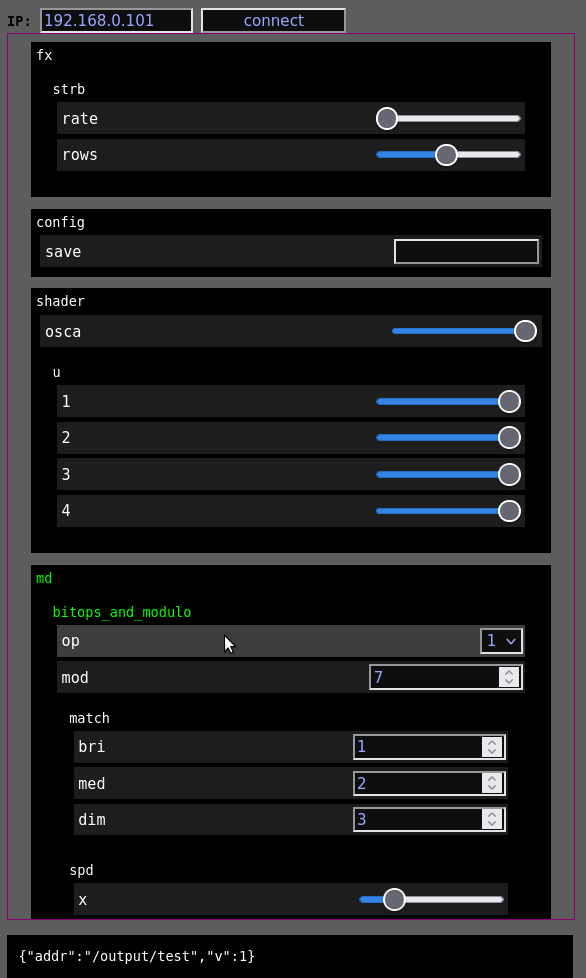
here is a simple example that uses a toggle to turn a light on and off at a desiered brightness.
// abbreviated Param example
#include "Param.h"
#define PWM_PIN 11
IntParam brightnessParam;
BoolParam lightSwitchParam;
ParamCollector paramCollector;
void setup(void){
// setup int param with osc style address and min/max/default.
brightnessParam.set("/light/brightness", 0, 255, 127);
brightnessParam.saveType = SAVE_ON_REQUEST;
// setup the boolean param
lightSwitchParam.set("/light/brightness", 0);
lightSwitchParam.setCallback(lightSwitchCallback);
lightSwitchParam.saveType = SAVE_ON_REQUEST;
// collect parameters
paramCollector.add(&brightnessParam);
paramCollector.add(&lightSwitchParam);
// initialise websocket server
paramWebsocketInit(¶mCollector);
}
void lightSwitchCallback(bool b){
// use parameter value with .v
analogWrite(PWM_PIN, b ? brightnessParam.v : 0);
}
void loop(){
// check for websocket messages
updateParamWebsocket();
}